Python Development
Description
Apex has integrated python 3.7 with which you can integrate your own applications.
# Example:
syrus4g@syrus-867698040023502 ~
$ python3
Python 3.7.7 (default, Mar 7 2022, 21:29:46)
[GCC 8.3.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> import os
>>> print ( os.getcwd() )
/data/users/syrus4g
>>> exit()
syrus4g@syrus-867698040023502 ~
Package Installation
To start using Python to develop applications you'll want to add pip to install python packages. To do this you need to add the 'dev_repo' on the device using the apx-core tool.
sudo apx-core add_dev_repo
And then install the python package installer, this will take a few minutes
sudo apx-core install python3-pip
Once that's done you can install packages with the following command:
pip3 install <PACKAGE_NAME> --user
Example use of requests package:
# Example
pip3 install requests --user
# Example
$ python3
>>> import requests
>>> x = requests.get('https://w3schools.com/python/demopage.htm')
>>> print(x.text)
<!DOCTYPE html>
<html>
<body>
<h1>This is a Test Page</h1>
</body>
</html>
>>>
Apex Message Broker & Database
Apex runs two Redis instances that can be used as message brokers or databases, the first one (User Application Redis) runs on the default port 6379 and the second one (System/Core Redis) is dedicated to Apex core applications and runs on port 7480.
Usage
# Example
pip3 install redis --user
System-Core Instance
This is a simple example of how to use the System-Core Redis instance to work with the database (GET, HGET, HGETALL, LRANGE) or the message broker (PSUBSCRIBE, SUBSCRIBE, MONITOR) for consults in your applications.
You can download and run some examples from here: Apex Core Python Examples
To see what data is available in APEX for your request, please go to Apex Message Broker & Database
Database consult example:
import redis
# Create a connection to the Redis server
redis_host = 'localhost'
redis_port = 7480
redis_password = None
# Create a Redis client
redis_client = redis.StrictRedis(host=redis_host, port=redis_port, password=redis_password, decode_responses=True)
# Testing GET command:
# GET: Retrieve and print the value of previous key
value = redis_client.get('network_interface')
print(f"Network Interface: {value}")
Message Broker listener example:
import redis
from time import sleep
# Create a connection to the Redis server
redis_host = 'localhost'
redis_port = 7480
redis_password = None
# Initialize redis connection
r = redis.StrictRedis(host=redis_host, port=redis_port, password=redis_password)
p = r.pubsub()
# To subscribe to one o multiple channels.
# In this case we will to subscribe to the interface pattern channel here you will receive
# all related to the I/O's
p.psubscribe("interface/*")
# Main loop function.
while True:
message = p.get_message()
if message:
print(f"Channel: {message['channel']}, Received message: {message['data']}")
sleep(0.01)
User Instance
This is a simple example of how to use the User Redis instance to work with the database (GET, SET, HGET,...) or the message broker (PUBLISH, PSUBSCRIBE,...) in your applications.
You can download and run some examples from here: Apex User Python Examples
Database consult example:
import redis
from time import time
# Create a connection to the Redis server
redis_host = 'localhost'
redis_port = 6379
redis_password = None
# Create a Redis client
redis_client = redis.StrictRedis(host=redis_host, port=redis_port, password=redis_password, decode_responses=True)
# Testing SET & GET commands:
# SET
current_time = str(int(time()))
redis_client.set('last_request_time', current_time)
# GET: Retrieve and print the value of previous key
value = redis_client.get('last_request_time')
print(f"Storing last request time: {value}")
Message Broker publish example:
import redis
from time import sleep, time
# Create a connection to the Redis server
redis_host = 'localhost'
redis_port = 6379
redis_password = None
# Create a Redis client for publishing
redis_publisher = redis.StrictRedis(host=redis_host, port=redis_port, password=redis_password)
# Publish messages to the channel
channel = 'notification/request'
for x in range(3):
current_time = str(int(time()))
message = "New request at " + current_time
print(f"Publish: {message}")
redis_publisher.publish(channel, message)
sleep(2)
Apex System Tool Execution
You can use the package os
to run apx commands or system-tools on the device.
import time
import os
# Send a message to a SyrusJS instance to set a variable
os.system("syrus-apps-manager send-message __cloud_syrusjs "set variable var_foo 1")
# Get the current time as a timestamp
current_timestamp = int(time.time())
# Format your command with the new timestamp
command = f"sudo apx-video create_clip --name={current_timestamp}_crash --time_win=-7,+7"
# Execute the command
os.system(command)
Build APEX Application
To build an application to be executed in apex you must create a compressed file as .zip in which you must add the following files, the binary or executable of your application, in the case the .py, a package.json file with the description of the application and an init.sh file that will have the definition of how your application will be executed.
Example s4test_app.zip content:
- init.sh
- package.json
- myapp.py
Make sure init.sh is executable
Before uploading an application to the Syrus 4, make sure the file
init.sh
is executable so that the Syrus 4 default user can execute it.This command is executed locally on your computer outside of the Syrus 4, before the file is compressed.
# Make file executable with chmod command chmod +x init.sh
The following is a basic example of the content that these files should contain:
init.sh
python3 test.py
package.json
{
"name": "myapp",
"version": "0.1.1",
"description": "My App application",
"icon": "https://myicons.com/logo.png",
"minApexVersion": "23.11.1",
"repository": "https://myrepo.com/applications/mmyApp/",
"author": "[email protected]"
}
test.py
print("Hello World")
Check applications state
If you need to consult if your app is running, installed, within an instance run any of this command from cloud or console.
Running apps
syrus-apps-manager get-running
Installed apps
syrus-apps-manager list-apps
App within an instance
syrus-apps-manager list-instances
Install & Run your Applications
To install and run your applications you need to use the apex tool syrus-apps-manager or the Management Tool as follows:
Option 1: Syrus Cloud (Recomended)
Once you are logged in the Syrus Cloud go to Applications -> Applications Tap
Click on Define Instance
Click on Application File and upload your myapp.zip
Change the instance name and complete the other fields if you want (Optional).
Add the devices to this instance and save, the application will run automatically.
Option 2: syrus-apps-manager
Copy your "myapp.zip" file to the Syrus4G device using SSH or Syrus Cloud.
Run
syrus-apps-manager install-app path_to_your_app
# Example
syrus-apps-manager install-app /data/users/syrus4g/myapps/myapp.zip
Run
syrus-apps-manager create-instance instace_name app_name app_version
# Example
syrus-apps-manager create-instance myinstace myapp 1.0.0
Run
syrus-apps-manager start instace_name
# Example
syrus-apps-manager start myinstace
Option 2: Management Tool
Once logged into the Management Tool go to Applications Manager
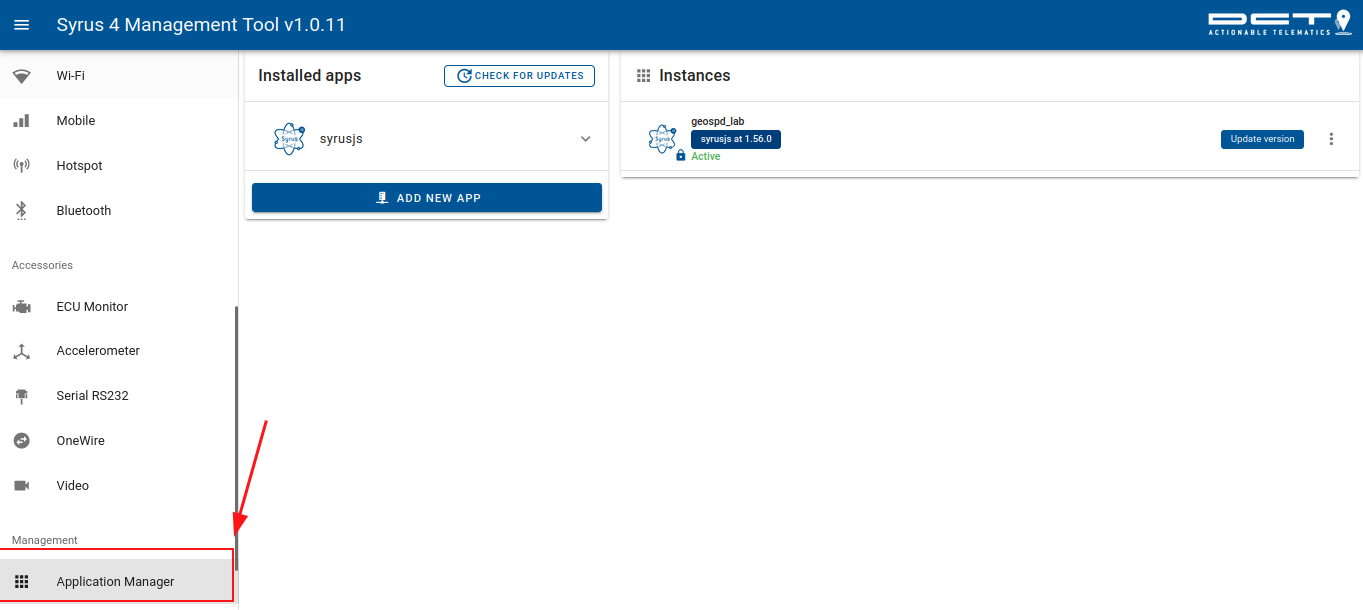
Click on ADD NEW APP and browse for the .zip file on your computer.
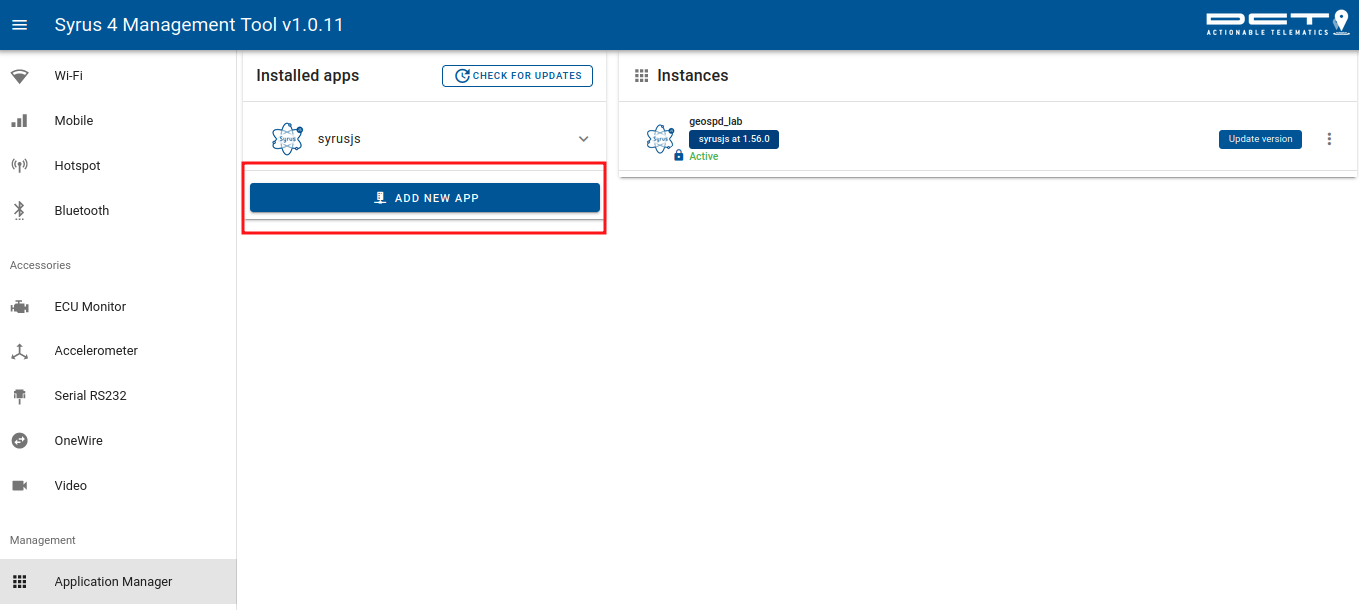
Click on INSTALL
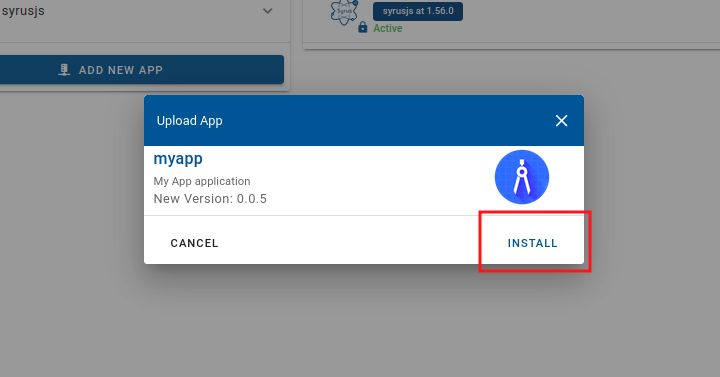
Click on the installed app and click on create instance and set the instance name.
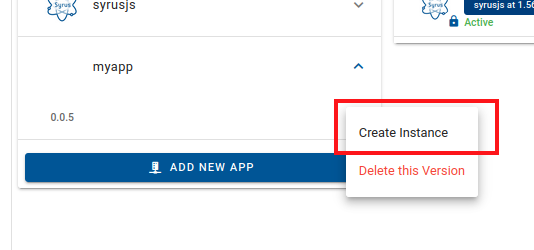
Once the instance is created click on the three points icon and start the instance.
Updated 10 months ago